목록콤퓨타 왕기초/JS (11)
파게로그
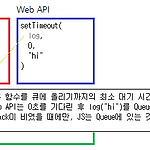
const result = add(10, 20); const add = (a, b) => a+b; console.log(result); // error JS 개발자 사이에서는 하나의 성경과 같은 것을 발견했다. https://github.com/leonardomso/33-js-concepts 한국어 버전은 이건데, 번역이 되어 있는 건 아니고 영어로 된 영상만 링크를 건 것 같다. https://github.com/yjs03057/33-js-concepts 어쨌든, 몇 가지 헷갈리는 개념들이 있어서 정리해 보았다. #1. undefined vs null vs NaN undefined: not defined yet null: defined as 'not exist' NaN: not a number let ..
위치 얻어오기(https://developer.mozilla.org/ko/docs/Web/API/Geolocation_API) navigator.geolocation.getCurrentPosition() 날씨 API(https://openweathermap.org/) async programming with Promise Promise (https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Promise) Using promises (https://wiki.developer.mozilla.org/ko/docs/Web/JavaScript/Guide/Using_promises) function getWeather(coord..
localStorage에는 String만 저장되므로 JS의 object 등을 저장하거나 불러올 때에는 JSON 메서드를 이용해 stringify 또는 parse해야 한다. const newArr = ["elem1", "elem2", "elem3", "elem4", "elem5"]; const newInt = 5; const newObj = { newArr, newInt }; const stringified = JSON.stringify(newObj); localStorage.setItem(localStorageAttribute, stringified); const localStorageValue = localStorage.getItem(localStorageAttribute); const parsed =..
element 생성 // 리스트 하나를 생성하는 경우 // 1. element 생성 const newUl = document.createElement("ul"), newLi1 = document.createElement("li"), newLi2 = document.createElement("li"); // 2. li를 ul에 붙임 newUl.appendChild(newLi1); newUl.appendChild(newLi2); // 3. ul을 필요한 곳에 붙임 document.querySelector("body").appendChild(newUl) element 제거 // 예를 들어, li 안에 delBtn이 있고, 이를 누르면 li가 지워짐 function handleDelBtnClick(event) ..
const arr1 = []; // Array.prototype.filter() // 지정한 콜백의 반환 결과가 true인 요소만 모은 새로운 배열을 반환합니다. const arr2 = arr1.filter(foo1); const arr3 = arr1.filter(function(element) { // ... }); // lambda 함수처럼 이름 없는 함수 사용 가능 // Array.prototype.forEach() // 배열의 각각의 요소에 대해 콜백을 호출합니다. arr1.forEach(foo2); arr1.forEach(function(element) { // ... }); Array에 적용할 수 있는 메서드 https://developer.mozilla.org/ko/docs/Web/JavaS..
const hi1 = document.querySelector("#hi1"); // const hi1 = document.getElementById("hi1"); const BASE_COLOR = "rgb(52, 73, 94)"; const OTHER_COLOR = "#7f8c8d"; var cnt = 0; function handleClick() { const currentColor = hi1.style.color; if (currentColor == BASE_COLOR) { hi1.style.color = OTHER_COLOR; } else { hi1.style.color = BASE_COLOR; } } function handleMouseenter() { const currentText = hi1...
event handler의 예시 function handleResize() { console.log("I have been resized."); } /* function handleResize() { console.log(event); } window.addEventListener("resize", handleResize); // function is called when an event occurs function handleClick() { title.style.color = "red"; } title.addEventListener("click", handleClick);
DOM: Document Object Model https://wit.nts-corp.com/2019/02/14/5522 간략하게 설명하자면, JS가 HTML의 모든 elements를 가져와서 이를 object로 바꾸어준 것이다. 몇 가지를 알 수 있는 간략한 코드... console.log(document) // document는 전체 HTML document.title = "New Title!"; const title1 = document.getElementById("title"); const title2 = document.querySelector("#title"); title.innerHTML = "Hi, from JS!"; title.style.color = "red"; // console.di..